Machine studying is a key area of Synthetic Intelligence that creates algorithms and coaching fashions. Two essential issues that machine studying tries to cope with are Regression and Classification. Many machine Studying algorithms carry out these two duties. Nevertheless, algorithms like Linear regression make assumptions in regards to the dataset. These algorithms might not work correctly if the dataset fails to fulfill the assumptions. The Determination Tree algorithm is unbiased of such assumptions and works wonderful for each regression and classification duties.
On this article, we are going to focus on the Determination Tree algorithm, and the way it works. We can even see easy methods to implement a call tree in Python, and its functions in several domains. By the top of this text, you’ll have a complete understanding of the choice bushes algorithm.
About us: Viso Suite is the pc imaginative and prescient infrastructure permitting enterprises to handle your complete software lifecycle. With Viso Suite, it’s doable for ML groups supply knowledge, practice fashions, and deploy them wherever, leading to simply 3 days of time-to-value. Study extra with a demo of Viso Suite.
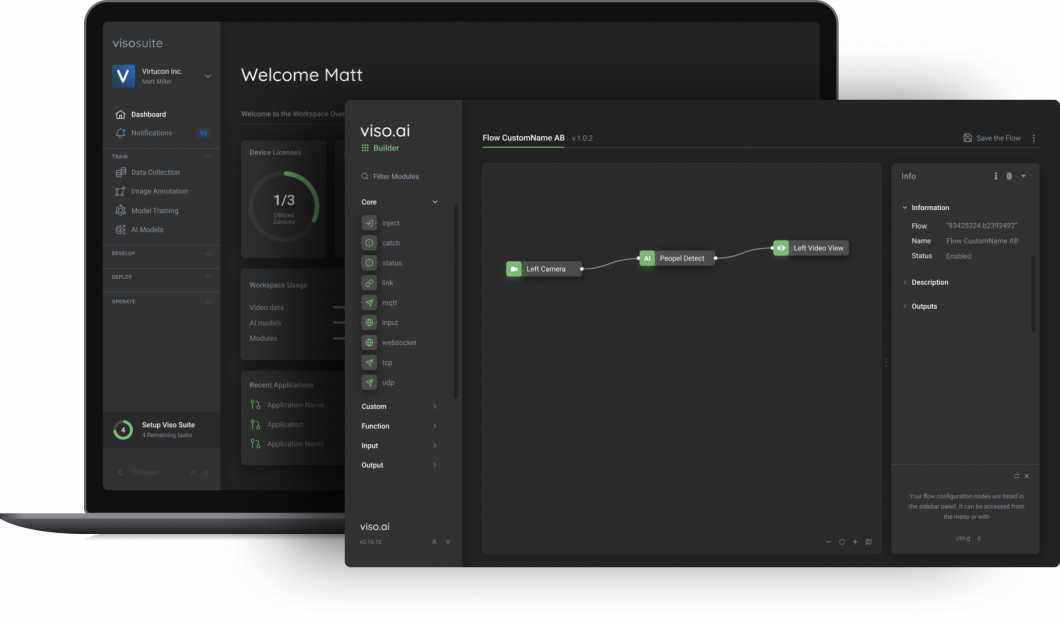
What’s a Determination Tree?
Determination Tree is a tree-based algorithm. Each classification and regression duties use this algorithm. It really works by creating bushes to make selections primarily based on the chances at every step. That is known as recursive partitioning.
It is a non-parametric and supervised studying algorithm. It doesn’t make assumptions in regards to the dataset and requires a labeled dataset for coaching. It has the construction as proven beneath:
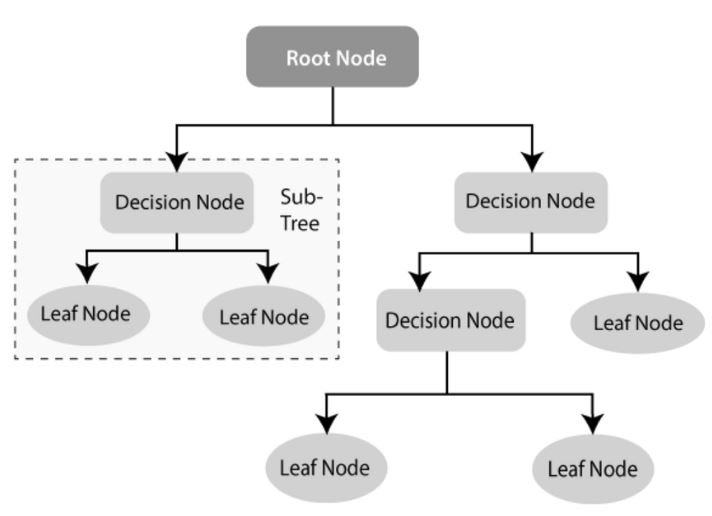
As we are able to see within the above tree diagram construction, the Determination tree algorithm has a number of nodes. They’re categorised as beneath.
- Root Node: The choice tree algorithm begins with the Root Node. This node represents the entire dataset and provides rise to all different nodes within the algorithm.
- Determination Node/Inner Node: These nodes are primarily based on the enter options of the dataset and are additional cut up into different inside nodes. Generally, these will also be known as father or mother nodes in the event that they cut up and provides rise to additional inside nodes that are known as baby nodes.
- Leaf Node/Terminal Node: This node is the top prediction or the category label of the choice tree. This node doesn’t cut up additional and stops the tree execution. The Leaf node represents the goal variable.
How Does a Determination Tree Work?
Think about a binary classification drawback of predicting if a given buyer is eligible for the mortgage or not. Let’s say the dataset has the next attributes:
Attribute | Description |
---|---|
Job | Occupation of the Applicant |
Age | Age of Applicant |
Revenue | Month-to-month Revenue of the Applicant |
Schooling | Schooling Qualification of the Applicant |
Marital Standing | Marital Standing of the Applicant |
Present Mortgage | Whether or not the Applicant has an present EMI or not |
Right here, the goal variable determines whether or not the shopper is eligible for the mortgage or not. The algorithm begins with your complete dataset because the Root Node. It splits the information recursively on options that give the very best info achieve.
This node of the tree provides rise to baby nodes. Timber characterize a call.
This course of continues till the standards for stopping is happy, which is set by the max depth. Constructing a call tree is a straightforward course of. The beneath picture illustrates the splitting course of on the attribute ‘Age’.
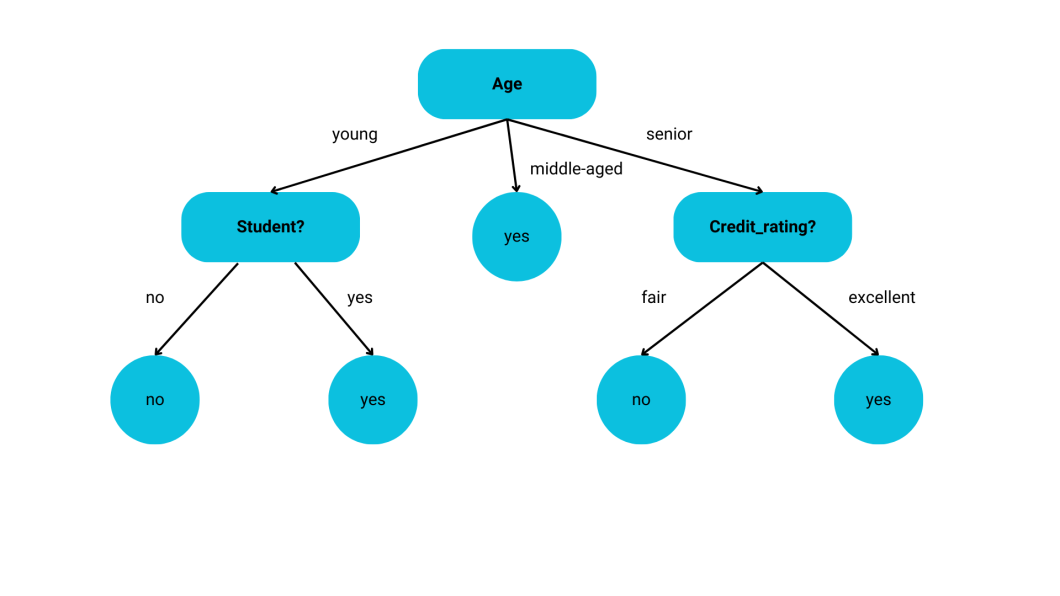
Completely different values of the ‘Age’ attribute are analyzed and the tree is cut up accordingly. Nevertheless, the standards for splitting the nodes must be decided. The algorithm doesn’t perceive what every attribute means.
Therefore it wants a worth to find out the standards for splitting the node.
Splitting Standards for Determination Tree
Determination tree fashions are primarily based on tree constructions. So, we’d like some standards to separate the nodes and create new nodes in order that the mannequin can higher determine the helpful options.
Info Achieve
- Info achieve is the measure of the discount within the Entropy at every node.
- Entropy is the measure of randomness or purity on the node.
- The system of Info Achieve is, Achieve(S,A) = Entropy(S) -∑n(i=1)(|Si|/|S|)*Entropy(Si)
- {S1,…, Si,…,Sn} = partition of S in keeping with worth of attribute A
- n = variety of attribute A
- |Si| = variety of instances within the partition Si
- |S| = whole variety of instances in S
- The system of Entropy is, Entropy=−∑i1=cpilogpi
- A node splits if it has the very best info achieve.
Gini Index
- The Gini index is the measure of the impurity within the dataset.
- It makes use of the chance distribution of the goal variables for calculations.
- The system for the Gini Index is, Gini(S)=1−∑pi2
- Classification and regression determination tree fashions use this criterion for splitting the nodes.
Discount in Variance
- Variance Discount measures the lower in variance of the goal variable.
- Regression duties primarily use this criterion.
- When the Variance is minimal, the node splits.
Chi-Squared Automated Interplay Detection (CHAID)
- This algorithm makes use of the Chi-Sq. check.
- It splits the node primarily based on the response between the dependent variable and the unbiased variables.
- Categorical variables akin to gender and shade use these standards for splitting.
A call tree mannequin builds the bushes utilizing the above splitting standards. Nevertheless, one essential drawback that each mannequin in machine studying is inclined to is over-fitting. Therefore, the Determination Tree mannequin can be susceptible to over-fitting. Generally, there are various methods to keep away from this. Probably the most generally used approach is Pruning.
What’s Pruning?
Timber that don’t assist the issue we are trying to resolve often start to develop. These bushes might carry out effectively on the coaching dataset. Nevertheless, they might fail to generalize past the check dataset. This leads to over-fitting.
Pruning is a method for stopping the event of pointless bushes. It prevents the tree from rising to its most depth. Pruning, in primary phrases, permits the mannequin to generalize efficiently on the check dataset, lowering overfitting.
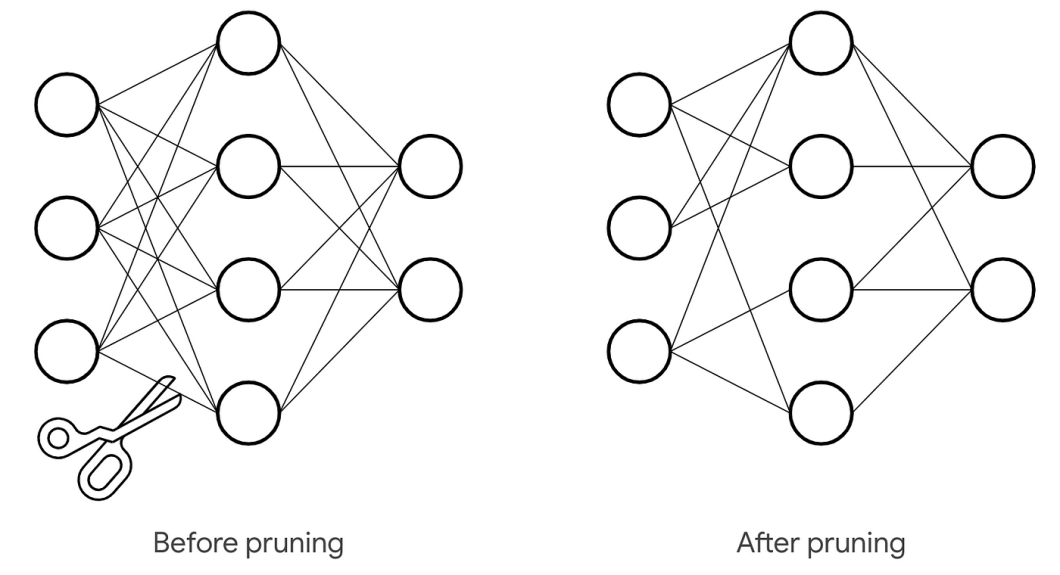
However how can we prune a call tree? There are two pruning strategies.
Pre-Pruning
This method entails stopping the expansion of the choice tree at early phases. The tree doesn’t attain its full depth. So, the bushes that don’t contribute to the mannequin don’t develop. That is often known as ‘Early Stopping’.
The expansion of the tree stops when the cross-validation error doesn’t lower. This course of is quick and environment friendly. We cease the tree at its early phases through the use of the parameters, ‘min_samples_split‘, ‘min_samples_leaf‘, and ‘max_depth‘. These are the hyper-parameters in a call tree algorithm.
Publish-Pruning
Publish-pruning permits the tree to develop to its full depth after which cuts down the pointless branches to stop over-fitting. Info achieve or Gini Impurity determines the standards to take away the tree department. ‘ccp_alpha‘ is the hyper-parameter used on this course of.
Price Complexity Pruning (ccp) controls the dimensions of the tree. The variety of nodes will increase with the rise in ‘ccp_alpha‘.
These are a few of the strategies to cut back over-fitting within the determination tree mannequin.
Python Determination Tree Classifier
We’ll use the 20 newsgroups dataset within the scikit-learn’s dataset module. This dataset is a classification dataset.
Step One: Import all the mandatory modules
from sklearn.datasets import fetch_20newsgroups from sklearn.feature_extraction.textual content import CountVectorizer from sklearn.tree import DecisionTreeClassifier from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score
Step Two: Load the dataset
# Load the 20 Newsgroups dataset newsgroups = fetch_20newsgroups(subset="all") X, y = newsgroups.knowledge, newsgroups.goal
Step Three: Vectorize the textual content knowledge
# Convert textual content knowledge to numerical options vectorizer = CountVectorizer() X_vectorized = vectorizer.fit_transform(X)
Step 4: Break up the information
# Break up the dataset into coaching and testing units X_train, X_test, y_train, y_test = train_test_split(X_vectorized, y, test_size=0.2, random_state=42)
Step 5: Create a classifier and practice
# Create and practice the choice tree classifier clf = DecisionTreeClassifier(random_state=42) clf.match(X_train, y_train)
Step Six: Make correct predictions on check knowledge
# Make predictions on check knowledge y_pred = clf.predict(X_test)
Step Seven: Consider the mannequin utilizing the Accuracy rating
# Consider the mannequin on the check set accuracy = accuracy_score(y_test, y_pred) print(f"Accuracy: {accuracy:.2f}")
The above code would produce a mannequin that has an ‘accuracy_score’ of 0.65. We will enhance the mannequin with hyper-parameter tuning and extra pre-processing steps.
Python Determination Tree Regressor
To construct a regression mannequin utilizing determination bushes, we are going to use the diabetes dataset accessible within the Scikit Study’s dataset module. We’ll use the ‘mean_squared_error‘ for analysis.
Step One: Import all the mandatory modules
from sklearn.datasets import load_diabetes from sklearn.tree import DecisionTreeRegressor from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error
Step Two: Load the dataset
# Load the Diabetes dataset diabetes = load_diabetes() X, y = diabetes.knowledge, diabetes.goal
This dataset doesn’t have any textual content knowledge and has solely numeric knowledge. So, there isn’t any must vectorize something. We’ll cut up the information for coaching the mannequin.
Step Three: Break up the dataset
# Break up the dataset into coaching and testing units X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 4: Create a Regressor and practice
# Create and practice the choice tree regressor reg = DecisionTreeRegressor(random_state=42) reg.match(X_train, y_train)
Step 5: Make correct predictions on check knowledge
# Make predictions on check knowledge y_pred = reg.predict(X_test)
Step 5: Consider the mannequin
# Consider the mannequin on the check set mse = mean_squared_error(y_test, y_pred) print(f"Imply Squared Error: {mse:.2f}")
The regressor will give a imply squared error of 4976.80. That is fairly excessive. We will optimize the mannequin additional through the use of hyper-parameter tuning and extra pre-processing steps.
Actual Life Makes use of Instances With Determination Timber
The Determination Tree algorithm is tree-based and can be utilized for each classification and regression tree functions. A Determination tree is a flowchart-like decision-making course of which makes it a straightforward algorithm to understand. In consequence, it’s utilized in a number of domains for classification and regression functions. It’s utilized in domains akin to:
Healthcare
Since determination bushes are tree-based algorithms, they can be utilized to find out a illness and its early analysis by analyzing the signs and check leads to the healthcare sector. They will also be used for remedy planning, and optimizing medical processes. For instance, we are able to examine the uncomfortable side effects and, the price of totally different remedy plans to make knowledgeable selections about affected person care.
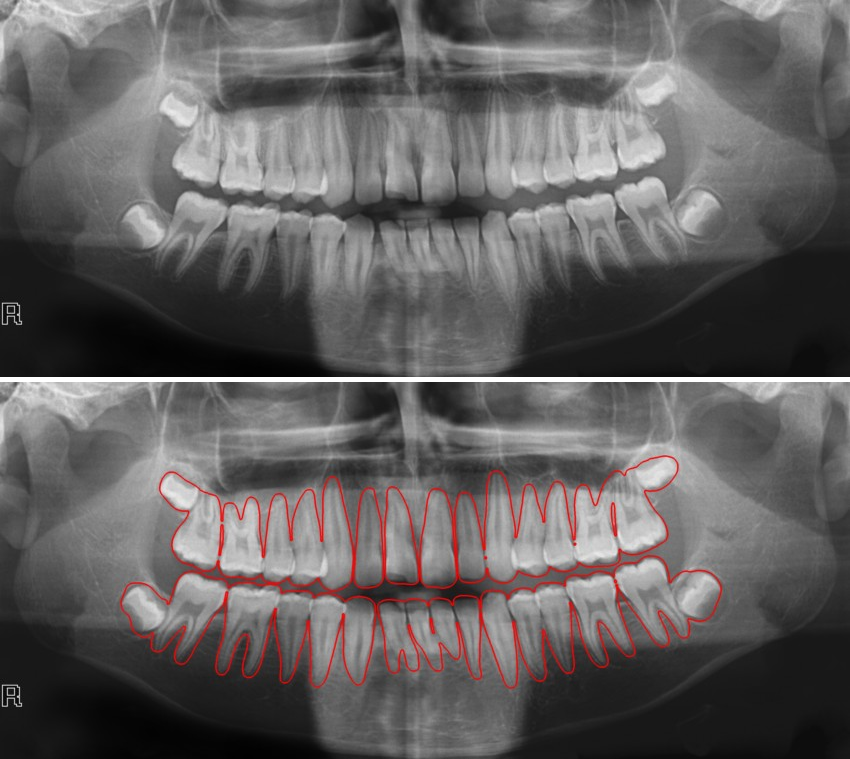
Banking Sector
Determination bushes can be utilized to construct a classifier for varied monetary use instances. We will detect fraudulent transactions, and mortgage eligibility of shoppers utilizing a call tree classifier. We will additionally consider the success of recent banking merchandise utilizing the tree-based determination construction.
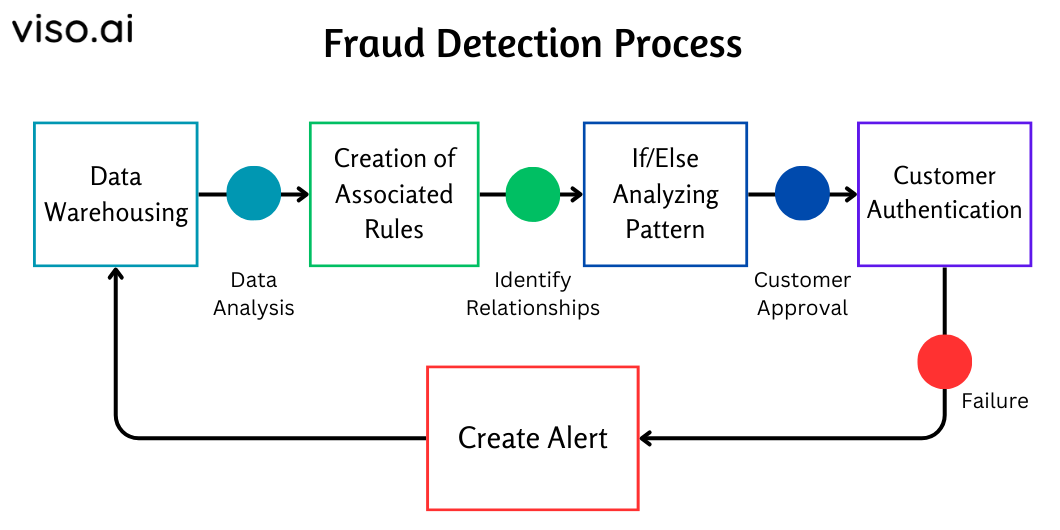
Danger Evaluation
Determination Timber are used to detect and manage potential dangers, one thing invaluable within the insurance coverage world. This permits analysts to contemplate varied eventualities and their implications. It may be utilized in venture administration, and strategic planning to optimize selections and save prices.
Knowledge Mining
Determination bushes are used for regression and classification duties. They’re additionally used for characteristic choice to determine vital variables and get rid of irrelevant options. They’re additionally used to deal with lacking values and mannequin non-linear relationships between varied variables.
Machine Studying as a discipline has developed in several methods. In case you are planning to study machine studying, then beginning your studying with a call tree is a good suggestion as it’s easy and simple to interpret. Determination Timber might be utilized with different algorithms utilizing ensembling, stacking, and staging which might enhance the efficiency of the mannequin.
What’s Subsequent?
To study extra in regards to the aspects of pc imaginative and prescient AI, try the next articles: